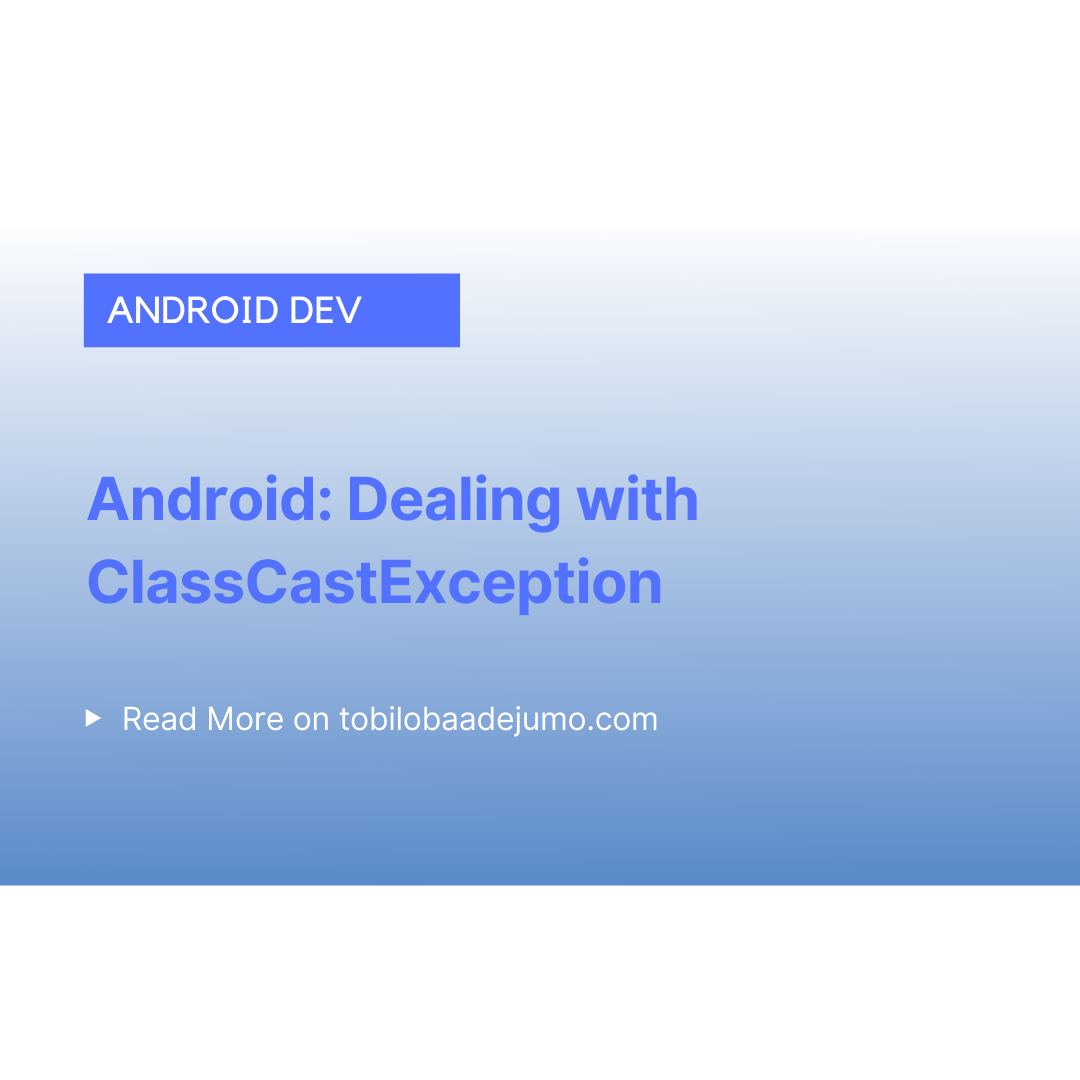
Android: Dealing with ClassCastException
ClassCastException is thrown to indicate that the code has attempted to cast an object to a subclass of which it is not an instance.
Dealing with exceptions in Android can be stressful, especially when the logcat messages are extremely verbose.
Apart from nullpointer exception all Android developers using Java have experienced π, there is also the classcast exception.
Iβll be explaining in details how to avoid this kind of runtime exception in Android.
What is ClassCast Exception?
From the documentation: βClassCastException is thrown to indicate that the code has attempted to cast an object to a subclass of which it is not an instance. β
What does this even mean?
Class Fruits {β¦}Class Banana extends Fruits {β¦}Class Apple extends Fruits {β¦}
Class Banana and Class Apple are both subclass of Class Fruit.
It is possible to say: Apple is a type of Fruit and it is also possible to pick a fruit at random with the intention of picking Apple and hope you picked Apple.
What do I even mean right? π
Let me explain again:
- Apple is a type of Fruit:
Apple apple = new Apple() ;Fruit fruit = (Fruit) apple;
- It is also possible to pick a fruit at random with the intention of picking Apple and hope you picked an Apple.
Fruit fruit = new Apple();Apple apple = (Apple) fruit;
But Apple is not a type of Banana and when you try casting Apple to Banana, it generates a ClassCastException. Also, when you pick a fruit at random with the intention of picking an Apple and you ended up picking a Banana, ClassCastException is generated. ClassCast Exception is a subclass of Runtime Exception. These exceptions are called unchecked exceptions because they are not checked by the compiler and are thrown by the Java Virtual Machine (JVM).
For example:
Banana banana = new Banana();Apple a = (Apple) banana; //generates ClassCastException.Fruit fruit = new Apple();Banana banana = (Banana) fruit; //generates ClassCastException
In order to avoid this kind of runtime exception in Android, it is advisable that you make use generics. What generics do is that whenever there is a classcast exception, it shows a compile time error and prevents you from running the code. This is quite clever while testing your code because the exception doesnβt wait to reveal itself when the application is put into production. Although, the use of generics has itβs downside but wouldnβt it be cool to have a compile time error rather than a runtime error? :)
Tobiloba Adejumo Newsletter
Join the newsletter to receive the latest updates in your inbox.